-
Mastering memory mapping in kdb+
19 June, 2025
-
KX named in AIFinTech100 list for solving AI’s real-time data challenges in financial services
18 June, 2025
-
Simultaneous search: How agentic AI searches smarter, not harder
16 June, 2025
-
How high-context analytics sharpen trading decisions and reduce risk
12 June, 2025
-
Crypto analytics at scale: How B2C2 trades smarter in a 24/7 market
5 June, 2025
-
Mastering kdb+ compression: Insights from the financial industry
2 June, 2025
-
Agentic trading in capital markets: Where ethics, risk, and alpha collide
30 May, 2025
-
Competitive cognition: Why agile intelligence wins in a volatile world
23 May, 2025
-
The rise of the citizen data scientist: How GenAI could reshape data access in finance
22 May, 2025
-
Revolutionizing video search with multimodal AI
21 May, 2025
-
Streamline FX trading with KX Flow
19 May, 2025
-
GPU-accelerated deep learning: Architecting agentic systems
14 May, 2025
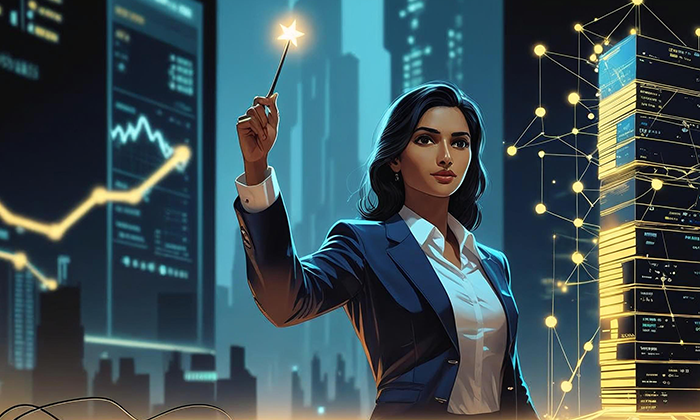
Market data magic with kdb Insights SDK
Discover how kdb Insights SDK helps developers deploy, scale, and manage real-time data pipelines for market data magic within a single, unified tech stack.